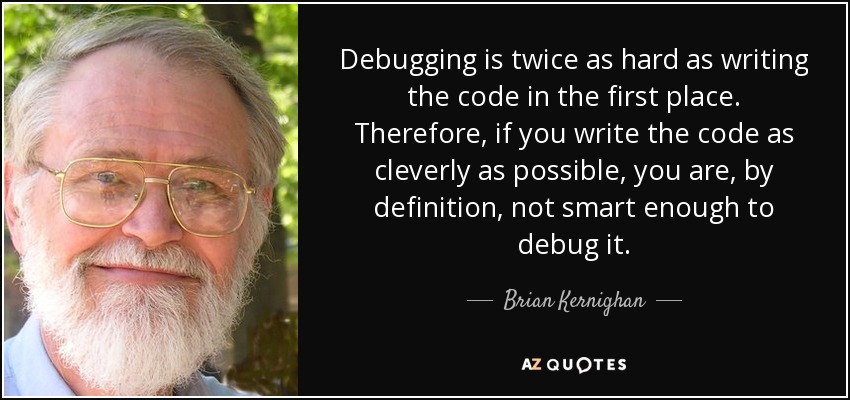
Before we start, I would like to mention that this article is not a tutorial on C programming language and you need to have some basic knowledge of C to understand the content.
String concatenation (C99)
Before compilation, the preprocessor will concatenate all the strings that are separated by whitespace.
#define DEBUG "[DEBUG] "
printf(DEBUG "facy error message goes here!\n");
// [DEBUG] fancy error message goes here!
Bit fields
Bit fields optimize memory usage when storing data that can be represented as a set of bits. They use integer types with a colon-specified number of bits, enabling the compiler to pack multiple bit fields into a single byte. However, implementation can be platform-dependent. Overall, bit fields reduce can memory usage and improve program efficiency but those are not necessary and should be avoided in today's world where compilers are smart and hardware is advanced.
struct {
unsigned int direction : 1;
unsigned int speed : 5;
unsigned int regen : 10;
} brake_flags;
Combining bit fields with unions can be useful for creating a compact data structure.
Below there a real example of bit fields that I've used back in the days when I was working at ITU Solar Car Team.
typedef union {
uint8_t data_u8[2];
struct {
unsigned int direction : 1;
unsigned int speed : 5;
unsigned int regen : 10;
} brake_flags;
unsigned int brake_data;
} BRAKE_DATA;
And then you can use it like this:
BRAKE_DATA brake_data;
brake_data.brake_flags.direction = 1;
brake_data.brake_flags.speed = 22;
brake_data.brake_flags.regen = 750;
CAN.write(brake_data.data_u8[0]);
CAN.write(brake_data.data_u8[1]);
Array indexing
In C, array indexing is equivalent to pointer arithmetic.
So the following two expressions yields to the same result:
array[index] = 5; // *(array + index) = 5;
index[array] = 5; // *(index + array) = 5;
So the following expression is true even though it looks weird ;)
array[0] == 0[array]; // true
Hinting compiler for branch prediction
I won't go into details about branch prediction¹ but in short, it's a technique used by modern processors to improve performance by guessing which branch in the code will be executed next.
In C, you can hint the compiler which branch is more likely to be executed (It's not guaranteed that the compiler will follow your hint, and discouraged to use it if you really don't know what you are doing)
if (__builtin_expect(x, 0)) {
// x is likely to be 0
}
if (__builtin_expect(x, 1)) {
// x is likely to be 1,
}
We can define a macro to make it more readable as it's used in the Linux kernel.
#define likely(x) __builtin_expect(!!(x), 1)
#define unlikely(x) __builtin_expect(!!(x), 0)
if (unlikely(is_error_occurred)) {
// if probability of error is low on your system then this branch is unlikely to be executed
return EXIT_FAILURE;
}
¹ Why is processing a sorted array faster than processing an unsorted array?